JavaScript
Accordion
Accordion helps to place many items in a limited space and provide easy access to every item. In this way, user can navigate through the items at their own pace.
Dependencies
JavaScript
<script src="js/libs/bootstrap-collapse.2.2.1.min.js" type="text/javascript"> </script> <script src="js/libs/sniui.accordion.min.js" type="text/javascript"> </script>
More information about the library can be found at Twitter Bootstrap.
Usage
Anim pariatur cliche reprehenderit, enim eiusmod high life accusamus terry richardson ad squid. 3 wolf moon officia aute, non cupidatat skateboard dolor brunch.
Anim pariatur cliche reprehenderit, enim eiusmod high life accusamus terry richardson ad squid. 3 wolf moon officia aute, non cupidatat skateboard dolor brunch.
Anim pariatur cliche reprehenderit, enim eiusmod high life accusamus terry richardson ad squid. 3 wolf moon officia aute, non cupidatat skateboard dolor brunch.
Anim pariatur cliche reprehenderit, enim eiusmod high life accusamus terry richardson ad squid. 3 wolf moon officia aute, non cupidatat skateboard dolor brunch.
<div class="accordion" id="accordion-parent"> <div class="accordion-group"> <div class="accordion-toggle accordion-selected" data-toggle="collapse" data-target="#collapseOne" data-parent="#accordion-parent"> <i class="icon12-previous"></i> <a> Lorem Ipsum </a> </div> <div id="collapseOne" class="accordion-body collapse in"> <div class="accordion-inner"> Anim pariatur cliche reprehenderit.... </div> </div> </div> </div>
Including data-attributes
To assign control of the collapsible element, provide data-target
attribute to the control element with the CSS selector of corresponding collapsible element.
Provide data-parent
attribute to the control element, with the CSS selector of the corresponding parent element, to get the Accordion like group management.
<div id="accordion-parent"> <div data-target="#collapseOne" data-parent="#accordion-parent"> </div> <div id="collapseOne"> </div> </div>
Getting Default Open Panel
Make sure to add the class collapse
to the collapsible element and class in
if you would like it open by default. Provide accordion-selected
class to control element which has the collapsible element expanded by default, to get the header styles.
Make sure to add accordion-toggle
class to control element as the header styles are handled through this class.
<div class="accordion-toggle accordion-selected"> </div> <div id="collapseOne" class="accordion-body collapse in"> </div>
Initialization
Accordion behavior can be implemented in two ways.
Through data-attributes
Bootstrap Collapse library uses data-toggle
attribute to get the collapsible behavior. All the control elements are provided with data-toggle="collapse"
attribute to get Accordion behavior.
<div data-toggle="collapse"> </div>
Through JavaScript
User needs to call collapse() on the collapsible element which needs to be expanded.
$("#collapseOne").collapse();
Action menu
The action menu can be used to display links to the user in a small, compact way. It's often used with table rows to allow users to perform actions on that row.
Space is automatically added to the right of icons embedded in the action panel links; there is no need to use the
.inline
helper class.
Simply include the Twitter Bootstrap drop down library (bootstrap-dropdown.2.0.4.min.js
) to implement the
drop down behavior. The library will look for anchors with an attribute of data-toggle="dropdown"
and
automatically add the required behavior. More information about the library can be found on
Twitter Bootstrap.
Use the .pullRight
class to right align the drop down menu.
Column 1 | Column 2 | Column 3 | |
---|---|---|---|
Value 1 | Value 2 | Value 3 |
<div class="actionMenu"> <a class="actionMenu-toggle" data-toggle="dropdown" href="#">Actions<b class="caret"></b></a> <ul class="actionMenu-menu" role="menu"> <li><a href="#"><i class="icon16-approve"></i>Action 1</a></li> <li><a href="#"><i class="icon16-approve"></i>Action 2</a></li> <li><a href="#"><i class="icon16-approve"></i>A really really long action</a></li> </ul> </div>
Auto Complete
Autocomplete helps users to locate the desired item in fewer steps by reducing the number of keystrokes and allowing faster user input.
Dependencies
JavaScript
<script src="js/libs/jquery-ui-1.9.2.custom.min.js" type="text/javascript"></script>
CSS
<link rel="stylesheet" href="css/jquery-ui/jquery-ui-1.9.2.custom.min.css" />
Usage and Initialization
Use the .autocomplete() function to convert a standard HTML input into a autocomplete dropdown. Add a input
with some id or class and initialize the JavaScript function autocomplete() with an array as data source using jQuery, targetting the element using the id or class.
More information about the jquery-ui autocomplete can be found at jquery-ui autocomplete.
<form class="form"> <input id="autocomplete"/> </form>JavaScript
$("#autocomplete").autocomplete({ source: availableTags });
Automatic Inline Help
Document your interface in-line with descriptive help blocks that automatically appears and disappears as the user navigates through the fields. The user will be provided with inline text related to the field that is clicked on automatically.
Dependencies
JavaScript
<script src="js/libs/bootstrap-popover.2.1.1.min.js" type="text/javascript"> </script> <script src="js/libs/sniui.auto-inline-help.min.js" type="text/javascript"> </script>
More information about the library can be found at Twitter Bootstrap.
Usage and Initialization
Automatic Inline Help can be initiated using the JavaScript method autoinline()
on the target element. The data to be displayed in the tool-tip is provided using the attribute data-content
<input type="text" id="emailid" data-content="You must supply a valid email address. We will not sell or disclose your email address to third parties"></input><br>JavaScript
$(function () { $("#emailid").autoinline(); });
Calendar
Calendar control displays a calendar from which the user can select a date.
Dependencies
JavaScript
<script src="js/libs/jquery-1.8.3.min.js" type="text/javascript"> </script> <script src="js/libs/jquery-ui-1.9.2.custom.min.js" type="text/javascript"> </script> <script src="js/libs/jquery.wijmo-open.all.2.3.1.min.js" type="text/javascript"> </script>
CSS
<link rel="stylesheet" href="css/jquery-ui/jquery-ui-1.9.2.custom.min.css" /> <link rel="stylesheet" href="css/wijmo/jquery.wijmo-complete.all.2.3.2.min.css" /> <link rel="stylesheet" href="css/bootstrap/bootstrap.css" />
Usage and Initialization
Use the .wijcalendar() function to convert a standard HTML div or span into a calendar.
More information about the wijmo calendar library can be found at wijmo calendar.
<div id="calendarControl"> </div>Javascript
$("#calendarControl").wijcalendar();
Date Picker
Date Picker is used to select a date. The selection can be made by clicking on the calendar icon which enables us to navigate to the required date and select a particular date. By default, current date is highlighted in the calendar.
Dependencies
JavaScript
<script src="js/libs/jquery.wijmo-open.all.2.3.1.min.js" type="text/javascript"> </script> <script src="js/libs/jquery.wijmo-complete.all.2.3.2.min.js" type="text/javascript"> </script> <script src="js/libs/sniui.wijmo.wijinputdate.min.js" type="text/javascript"> </script>
Usage and Initialization
Date Picker control is dependent on Wijmo library (which is in turn dependent on jqueryUI library). Use the inputdate() function to convert a standard HTML div or span into a datepicker. Add a div
or span
with some id or class and initialize the JavaScript function inputdate() using jQuery, targetting the element using the id or class. By default showTrigger
value is false in Wijmo. It is set to true to make the calendar button default open.
More information about the library can be found at Wijmo Date Picker.
<input type="text" id="datePicker" />JavaScript
$(function () { $('#datePicker').inputdate({ showTrigger: true, dateFormat: 'd', showNullText: true, nullText: "mm/dd/yyyy" }); });
Date Picker Range
minDate
and maxDate
can be used to restrict the selectable dates as seen in the example below. More information about min and max date can be found at Wijmo Date Picker min and max.
<input type="text" id="datePickerRange" />JavaScript for Date Picker Range
$(function () { $("#datePickerRange").inputdate({ minDate: '01/05/2013', maxDate: '12/06/2013', }); });
Determinate Progress Bar
This component can be used to show the percentage of completion of a process.
Dependencies
JavaScript
<script src="js/libs/jquery-ui-1.9.2.custom.min.js" type="text/javascript"> </script> <script src="js/libs/sniui.determinate-progressbar.min.js" type="text/javascript"> </script>
More information about the library can be found at jquery ui progressbar.
Usage & Initialization
Determinate Progress Bar can be initiated using the JavaScript method determinateProgressBar()
on the target element. The attribute data-content
is used to display the content in the data label of the progress bar.
<div id="determinateProgressBarGreen" data-content="data displayed through data-attributes"> </div>
Initialize the progress bar with value
option specified.
$( "#determinateProgressBarGreen" ).determinateProgressBar({ value: 60 });
Options associated with Determinate Progress Bar
color
The color of the indicator by default is green. The user has the option to change it to blue through color
option.
content
This option allows user to change the data label's content through JavaScript.
$( "#determinateProgressBarBlue" ).determinateProgressBar({ value: 60, color: "blue", content: "data displayed through content option" });
Note: Information provided through content
option overrides the value provided through data-content
attribute.
Events associated with Determinate Progress Bar
change()
Triggered when the value of the progress bar changes.
complete()
Triggered when the value of the progress bar reaches the max value.
$( "#determinateProgressBar" ).determinateProgressBar({ change: function( event, ui ) {}, complete: function( event, ui ) {} });
Dialog
Dialogs can be used to display popup windows without opening additional tabs or browser
windows. Just add a <div>
with the content that should be displayed in
in the dialog. The title
attribute can be used to control the title displayed
on the dialog.
jQuery UI provides the dialog functionality, but relies on the sniui.dialog.1.1.0.js
script to change the dialog buttons into links. Add the script reference after jQuery UI
at the end of your HTML document.
The "Example Dialog" link is initialized by the example JavaScript shown. For more information on configuration options, visit jQuery UI.
Test dialog
HTML
<div id="modalDialog" title="Modal Dialog"> ... </div>
JavaScript
$('#modalDialog').dialog({ autoOpen: false, buttons: { 'ok': { 'class': 'button button-primary', click: function () { $(this).dialog('close'); }, text: 'Save' }, 'cancel': { click: function () { $(this).dialog('close'); }, text: 'Cancel' } }, height: 200, modal: true, resizable: false });
Grid
The grid extends a basic table with advanced features like filtering, sorting and pagination. Simply start with some basic table HTML.
<table id="myTable" class="table"> <thead> <tr> <th>...</th> <th>...</th> </tr> </thead> <tbody> <tr> <td>...</td> <td>...</td> </tr> </tbody> </table>
# | First Name | Last Name | Username |
---|---|---|---|
1 | Mark | Otto | @mdo |
2 | Jacob | Thornton | @fat |
3 | Larry | the Bird | |
4 | Mark | Otto | @mdo |
5 | Jacob | Thornton | @fat |
6 | Larry | the Bird | |
7 | Mark | Otto | @mdo |
8 | Jacob | Thornton | @fat |
9 | Larry | the Bird | |
10 | Mark | Otto | @mdo |
11 | Mark | Otto | @mdo |
12 | Jacob | Thornton | @fat |
13 | Larry | the Bird | |
14 | Mark | Otto | @mdo |
15 | Jacob | Thornton | @fat |
16 | Larry | the Bird | |
17 | Mark | Otto | @mdo |
18 | Jacob | Thornton | @fat |
19 | Larry | the Bird | |
20 | Mark | Otto | @mdo |
21 | Mark | Otto | @mdo |
22 | Jacob | Thornton | @fat |
23 | Larry | the Bird | |
24 | Mark | Otto | @mdo |
25 | Jacob | Thornton | @fat |
26 | Larry | the Bird | |
27 | Mark | Otto | @mdo |
28 | Jacob | Thornton | @fat |
29 | Larry | the Bird | |
30 | Mark | Otto | @mdo |
31 | Mark | Otto | @mdo |
32 | Jacob | Thornton | @fat |
33 | Larry | the Bird | |
34 | Mark | Otto | @mdo |
35 | Jacob | Thornton | @fat |
36 | Larry | the Bird | |
37 | Mark | Otto | @mdo |
38 | Jacob | Thornton | @fat |
39 | Larry | the Bird | |
40 | Mark | Otto | @mdo |
41 | Mark | Otto | @mdo |
42 | Jacob | Thornton | @fat |
43 | Larry | the Bird | |
44 | Mark | Otto | @mdo |
45 | Jacob | Thornton | @fat |
46 | Larry | the Bird | |
47 | Mark | Otto | @mdo |
48 | Jacob | Thornton | @fat |
49 | Larry | the Bird | |
50 | Mark | Otto | @mdo |
51 | Mark | Otto | @mdo |
52 | Jacob | Thornton | @fat |
53 | Larry | the Bird | |
54 | Mark | Otto | @mdo |
55 | Jacob | Thornton | @fat |
56 | Larry | the Bird | |
57 | Mark | Otto | @mdo |
58 | Jacob | Thornton | @fat |
59 | Larry | the Bird | |
60 | Mark | Otto | @mdo |
61 | Mark | Otto | @mdo |
62 | Jacob | Thornton | @fat |
63 | Larry | the Bird | |
64 | Mark | Otto | @mdo |
65 | Jacob | Thornton | @fat |
66 | Larry | the Bird | |
67 | Mark | Otto | @mdo |
68 | Jacob | Thornton | @fat |
69 | Larry | the Bird |
Initialization
To get started with the grid, add the DataTables library (jquery.dataTables.1.9.3.min.js
). Use the .dataTable()
function to convert a standard HTML table into a full-featured grid.
The 'bFilter': false
and 'bLengthChange': false
options can be used to disable the built in
filter and page size controls.
$('#myTable').dataTable({ 'bFilter': false, 'bLengthChange': false });
Visit the DataTables website for more information about initialization options and advanced features of the control.
Column Sorting Indicators
The column sort indicators can be enabled by adding the SNI UI extensions for DataTables (sniui.dataTables.1.1.0.min.js
).
The extensions provide a callback to customize the header appearance when as the grid is drawn.
$('#myTable').dataTable({ 'fnHeaderCallback': Scripps.DataTables.fnHeaderCallback });
Pagination
The SNI UI extensions include a custom pagination plug in to get the desired appearance. Simply specify the
'scripps'
pagination appearance during initialization.
$('#myTable').dataTable({ 'sPaginationType': 'scripps' });
Indeterminate Progress Indicator
Indeterminate Progress Indicator can be used to let the user know that something is occurring or that the system is busy. This can be associated with actions for which we are not sure about the time required for completion.
Dependencies
JavaScript
<script src="js/libs/jquery-ui-1.9.2.custom.min.js" type="text/javascript"> </script> <script src="js/libs/sniui.indeterminate-progress-indicator.min.js" type="text/javascript"> </script>
Usage
Initialization
Indeterminate Progress indicator can be initialized by calling the widget constructor indeterminateProgressIndicator()
on the target element as shown in the javascript section.
We have the following events associated with this component which can be handled according to the requirement,
Event | Description |
---|---|
show |
Raised before the indicator has been made visible |
shown |
Raised after the indicator has been made visible |
hide |
Raised before the indicator is hidden |
hidden |
Raised after the indicator has been hidden |
The options available with this component are,
Option | Description |
---|---|
modal |
true - hides the parent using a transparent overlay; false - no overlay is used. Default value is false. |
position |
Object (Based on the jQuery UI position utility)my : Default value is center. at : Default value is center.
of : Default value is window.
|
zIndex |
The starting z-index for the dialog. Default value is 1000. |
Following are the methods responsible for showing and hiding this component,
Method | Description |
---|---|
show() |
to show the component |
hide() |
to hide the component |
<div id="indicator"></div> <input type="text" id="emailaddress" title="emailid title" ></input>JavaScript
$(function () { $("#indicator").indeterminateProgressIndicator({ //determines if the widget should be shown as modal or not modal: false, //default position of the widget position: { my: "left top", at: "right top", of: "#emailaddress" } }); $("#emailaddress").keyup(function(){ if($(this).val() == '') { $("#indicator").indeterminateProgressIndicator("hide"); } else { $("#indicator").indeterminateProgressIndicator("show"); } }); });
Multi Select Drop Down
Multi Select Drop Down component helps the user to select one or more values from a range of possible values.
Dependencies
JavaScript
<script src="js/libs/jquery-1.8.3.min.js" type="text/javascript"> </script> <script src="js/libs/jquery-ui-1.9.2.custom.min.js" type="text/javascript"> </script> <script src="js/libs/jquery.multiselect.js" type="text/javascript"> </script>
Usage and Initialization
Just provide the content for the Multi Select container as shown in the HTML section.
Use the .multiselect()
widget function to get the functionality of this component.
Below are the options that we can provide while triggering the component,
Option | Value | Description |
---|---|---|
selectedList |
100 |
The text to display in the select box when options are selected. Since every selected item should be shown in the select box, provide a higher value such as 100 for this option. |
noneSelectedText |
'' |
The default text in the select box when no options have been selected. |
checkAllText |
'Check All' |
The text of the "check all" link. |
Uncheck All |
'Uncheck All' |
The text of the "uncheck all" link. |
Visit the jquery UI Multiple Select Widget component website for more information about initialization options and advanced features of the component.
<select multiple="multiple"> <option value="item1">Item 1</option> <option value="item2">Item 2</option> <option value="item3">Item 3</option> <option value="item4">Item 4</option> <option value="item5">Item 5</option> <option value="item6">Item 6</option> <option value="item7">Item 7</option> </select>JavaScript
$("select").multiselect({ selectedList: 100, // 0-based index noneSelectedText: '', checkAllText: 'Check All', uncheckAllText: 'Uncheck All' });
Tabs
Tabs help to separate content into different panes. Tabs are arranged horizontally above the content, and the selected tab is highlighted while the panes can be viewed one at a time.
Dependencies
JavaScript
<script src="js/libs/jquery-1.8.3.min.js" type="text/javascript"> </script> <script src="js/libs/jquery-ui-1.9.2.custom.min.js" type="text/javascript"> </script>
Visit the jquery UI tabs component website for more information about initialization options and advanced features of the component.
Usage
Lorem ipsum dolar adipiscing elit. Donec quis purus vel libero bibendum adipis. Suspendisse ac vene justo. Sed ac consequat eros.
Label 2 content.
Label 3 content.
Label 3 content.
Label 4 content.
Label 5 content.
Initialization
Just provide class="inlineTabs"
for the tabs container and provide the content as shown in the HTML section.
Use the .tabs()
function to get the functionality of this tabs component. The JavaScript code to trigger the component can be seen in the JavaScript section.
<div class="inlineTabs"> <ul> <li><a href="#tabs-1">Label 1</a></li> <li><a href="#tabs-2">Label 2</a></li> </ul> <div id="tabs-1"> <p>Lorem ipsum dolar adipiscing elit. Donec quis purus vel libero bibendum adipis. Suspendisse ac vene justo. Sed ac consequat eros.</p> </div> <div id="tabs-2"> <p>Label 2 content.</p> </div> </div>JavaScript
$(function() { $( ".inlineTabs" ).tabs(); });
Tool Tip
This component helps to produce a tool tip on hovering over a particular target area. It is designed in such a way that, tool tip remains activated for the complete time the user has the mouser over the UI element or within the tooltip itself.
Dependencies
JavaScript
<script src="js/libs/bootstrap-popover.2.1.1.min.js" type="text/javascript"> </script> <script src="js/libs/sniui.tool-tip.min.js" type="text/javascript"> </script>
More information about the library can be found at Twitter Bootstrap.
Usage and Initialization
Tool Tip can be initiated by using the method .toolTip()
, however the target element needs the attributes title
and data-content
attributes to display the title and content of the tool tip respectively.
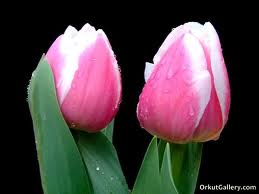
<img src="images/toolTipTarget.jpg" id="toolTipTarget" alt="" data-content="Lorem ipsum dolar adipiscing elit..." title="Lorem Ipsum"/>JavaScript
$(function () { $("#toolTipTarget").toolTip(); });
Customizing Position and Delay
data-placement
can be used to position the tool tip in different positions viz top, bottom, left and right by providing the value accordingly. By default, the tooltip is made to appear to the right of the target element.
The user can also set the delay in time for the tooltip to appear /disappear. This can be implemented by providing data-delay
attribute with the desired time limit (in ms). Default value provided is 300ms.
<img src="images/toolTipTarget.jpg" id="toolTipTarget" alt="" data-placement="top" data-delay="500"/>
Tree View
Tree View is used to view and select multiple branches and leaves in a hierarchical order. It can be used when there are many branches and can be expanded as needed by the user.
Dependencies
JavaScript
<script src="js/libs/jquery.wijmo-open.all.2.3.1.min.js" type="text/javascript"> </script> <script src="js/libs/jquery.wijmo-complete.all.2.3.2.min.js" type="text/javascript"> </script>
Usage and Initialization
Tree View is dependent on Wijmo library (which is in turn dependent on jqueryUI library). Use the .wijtree() function to convert a standard HTML unordered list into a treeView.
More information about the library can be found at Wijmo Tree View.
<ul id="tree"> <li class="folder"><a><span>Value 1</span></a> <ul> <li class="folder"><a><span>Child Value 1</span></a> <ul> <li class="file"><a><span>Child Value 1</span></a></li> <li class="file"><a><span>Child Value 2</span></a></li> <li class="file"><a><span>Child Value 3</span></a></li> <li class="file"><a><span>Child Value 4</span></a></li> <li class="file"><a><span>Child Value 5</span></a></li> </ul> </li> <li class="file"><a><span>Child Value 2</span></a></li> <li class="file"><a><span>Child Value 3</span></a></li> <li class="file"><a><span>Child Value 4</span></a></li> <li class="file"><a><span>Child Value 5</span></a></li> </ul> </li> </ul> </div>JavaScript
$(document).ready(function () { var tv = $("#tree").wijtree({ showCheckBoxes: true, allowEdit: true }); });
Options
Other Icons
To add customized icons, add the necessary class to the node (li
) by providing it in the method wijtreenode
as suggested by Wijmo TreeNode Options.
$(document).ready(function () { var tv = $("#otherIconTree").wijtree(); $("#otherIconTree li.folder").wijtreenode({collapsedIconClass : 'icon16-folder',expandedIconClass:'icon16-folder'});
$("#otherIconTree li.msword").wijtreenode({itemIconClass: 'icon16-msword'});
$("#otherIconTree li.xml").wijtreenode({itemIconClass: 'icon16-xml'}); });
User Activated Inline Help
User Activated Inline Help is used to display additional information which can be displayed as a popover, next to the element that is used as a trigger. The popover can be closed by clicking on the close link provided in it or by clicking anywhere outside it.
Dependencies
JavaScript
<script src="js/libs/bootstrap-popover.2.1.1.min.js" type="text/javascript"> </script> <script src="js/libs/sniui.userInlineHelp.min.js" type="text/javascript"> </script>
More information about the library can be found at Twitter Bootstrap.
Usage and Initialization
User Activated Inline Help can be initiated using the JavaScript method userInlineHelp()
on the target element. The attributes title
and data-content
are used to display the title and the message respectively in the popover.
<i id="userInlineHelp" class="icon12-question" data-content="Lorem Ipsum dolar sedit. Vestis Verim Redit. Manus Manum Lavat." title="Lorem Ipsum"></i>JavaScript
$(function (){ $('#userInlineHelp').userInlineHelp(); });